iOS SDK migration guide
This migration guide will inform about bigger changes in SDK api, that might affect you.
[8.6.2] -> [8.6.3]
Updated Face capture oval design
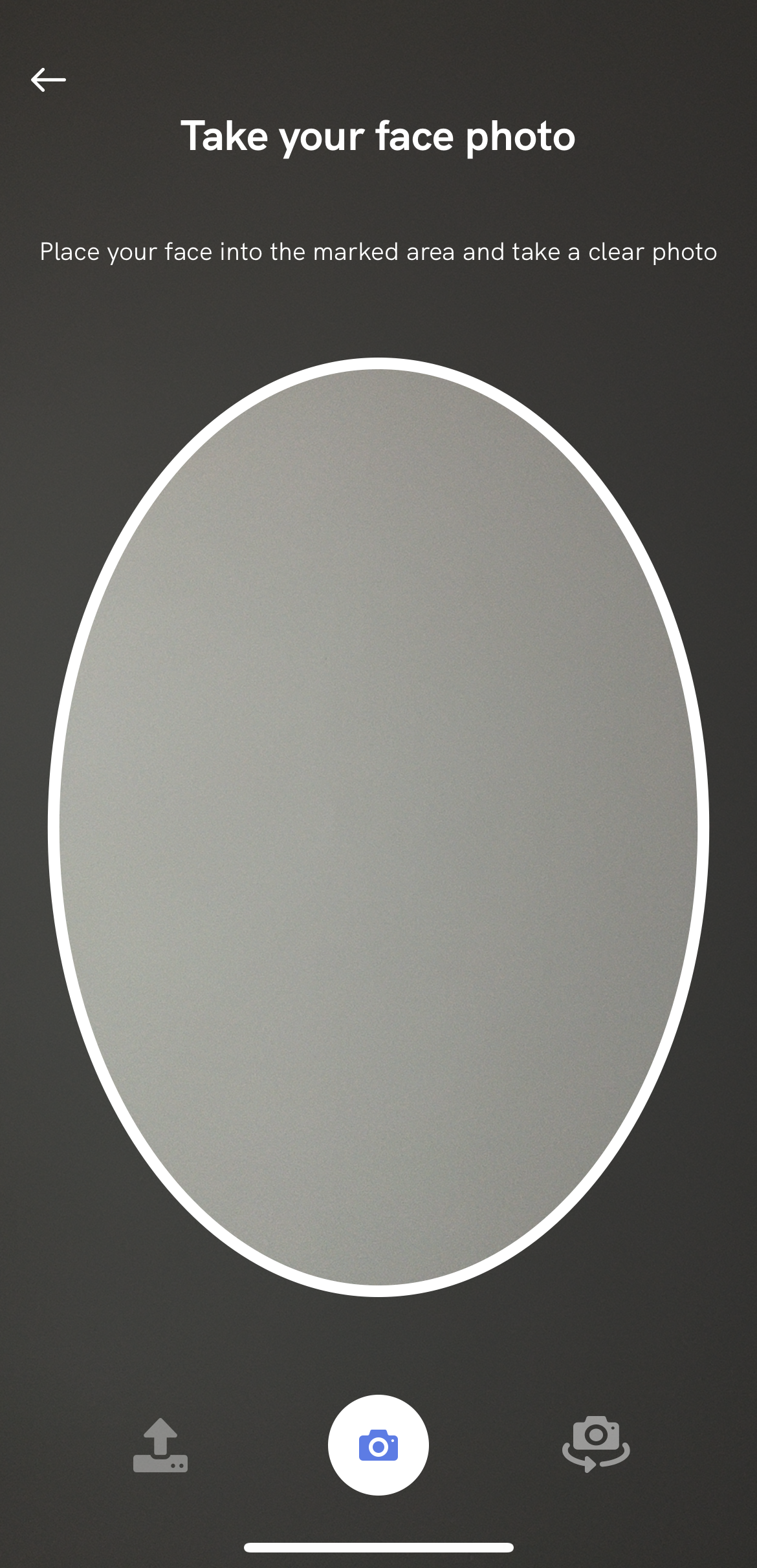
Please follow this migration guide point, if you have implemented custom views of FaceCameraViewableV2.
A new idenfyUIViewFaceOvalV3 oval class was added, which replaces the idenfyUIViewFaceOval in the FaceCameraViewableV2:
public var idenfyUIViewFaceOvalV3: IdenfyFaceOvalV3? = {
let view = IdenfyFaceOvalV3(frame: .zero)
view.translatesAutoresizingMaskIntoConstraints = false
view.isOpaque = false
return view
}()
func setupUIViewFaceOval() {
if let unwrappedIdenfyUIViewFaceOvalV3 = idenfyUIViewFaceOvalV3 {
addSubview(unwrappedIdenfyUIViewFaceOvalV3)
unwrappedIdenfyUIViewFaceOvalV3.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
unwrappedIdenfyUIViewFaceOvalV3.bottomAnchor.constraint(equalTo: cameraSessionsButtons.idenfyUIViewContainerOfButtons.topAnchor).isActive = true
let top = CameraSessionUIHelper.getDrawerHeight()
unwrappedIdenfyUIViewFaceOvalV3.topAnchor.constraint(equalTo: topAnchor, constant: top).isActive = true
unwrappedIdenfyUIViewFaceOvalV3.leftAnchor.constraint(equalTo: leftAnchor).isActive = true
unwrappedIdenfyUIViewFaceOvalV3.rightAnchor.constraint(equalTo: rightAnchor).isActive = true
unwrappedIdenfyUIViewFaceOvalV3.setNeedsDisplay()
}
}
The idenfyUIViewFaceOval is still available, however it is deprecated and will be removed in the future.
[8.5.x] -> [8.6.0]
Added realtime blur glare detection in document capture
Please follow this migration guide point, if you have implemented custom views of DocumentCameraViewableV2.
With the latest realtime blur glare detection feature, a warning alert card was added to the DocumentCameraViewableV2:
public var documentCameraAlertCard: UIView = {
let view = UIView(frame: .zero)
view.translatesAutoresizingMaskIntoConstraints = false
view.backgroundColor = IdenfyDocumentCameraSessionUISettingsV2.idenfyDocumentCameraAlertCardBackgroundColor
view.isHidden = true
return view
}()
public var documentCameraAlertCardImage: UIImageView = {
let imageView = UIImageView()
imageView.translatesAutoresizingMaskIntoConstraints = false
imageView.layer.masksToBounds = true
imageView.image = UIImage(named: "idenfy_ic_warning_alert", in: Bundle(identifier: "com.idenfy.idenfyviews"), compatibleWith: nil)?.withRenderingMode(.alwaysTemplate)
imageView.contentMode = .scaleAspectFit
imageView.tintColor = IdenfyDocumentCameraSessionUISettingsV2.idenfyDocumentCameraAlertCardImageTintColor
return imageView
}()
public var documentCameraAlertCardTitle: UILabel = {
let label = UILabel(frame: .zero)
label.translatesAutoresizingMaskIntoConstraints = false
label.numberOfLines = 0
label.font = IdenfyDocumentCameraSessionUISettingsV2.idenfyDocumentCameraAlertCardTitleFont
label.textAlignment = .left
label.textColor = IdenfyDocumentCameraSessionUISettingsV2.idenfyDocumentCameraAlertCardTitleColor
return label
}()
[8.5.x] -> [8.5.7]
KYC Questionnaire FILE and IMAGE questions are merged, therefore ImageQuestionCell is removed
.withImageQuestionCellView(ImageQuestionCell.self)
[8.4.x] -> [8.5.0]
Added Face Detection progress View to the camera drawer
Please follow this migration guide point, if you have implemented custom views of DrawerContentViewableV2.
With the latest face authentication auto capture feature, a progress view was added to the DrawerContentViewableV2:
public var faceDetectionProgressView: UIProgressView = {
let progressView = UIProgressView(frame: .zero)
progressView.translatesAutoresizingMaskIntoConstraints = false
progressView.progressTintColor = IdenfyInstructionAlertUISettigsV2.idenfyInstructionAlertProgressBarFillColor
progressView.trackTintColor = UIColor.clear
progressView.isHidden = true
return progressView
}()
...
open func setupProgressView() {
addSubview(faceDetectionProgressView)
faceDetectionProgressView.leftAnchor.constraint(equalTo: leftAnchor).isActive = true
faceDetectionProgressView.bottomAnchor.constraint(equalTo: bottomAnchor).isActive = true
faceDetectionProgressView.rightAnchor.constraint(equalTo: rightAnchor).isActive = true
faceDetectionProgressView.heightAnchor.constraint(equalToConstant: 3).isActive = true
}
[8.3.x] ~> [8.4.0]
Removed Dynamic OnBoarding view
.withOnBoardingViewType(.multipleDynamic) option is no longer available in the IdenfyUISettingsV2.
Please follow this migration guide point, if you have implemented custom views of DynamicCameraOnBoardingViewableV2.
The IdenfyViewsBuilderV2 will no longer have a .withDynamicCameraOnBoardingView option, please use .withStaticCameraOnBoardingView viewable.
You can check new, fully implemented views in our sample application here
The IdenfyDynamicCameraOnBoardingViewUISettingsV2 class has been removed as well. The static onBoarding view its own IdenfyStaticCameraOnBoardingViewUISettingsV2 class.
[8.2.x] ~> [8.3.0]
Removed instructions drawer option from camera view
Since the instructions drawer has been removed, the top drawer will remain static as if instructions were disabled. Now the drawer will only hold camera descriptions and the appbar.
.withInstructions(IdenfyInstructionsType.drawer) option is no longer available in the IdenfyUIBuilderV2.
8.2.x | 8.3.0 |
---|---|
![]() ![]() | ![]() |
Please follow this migration guide point, if you have implemented custom views of DrawerContentViewableV2, DocumentCameraViewableV2 or FaceCameraViewableV2.
The IdenfyViewsBuilderV2 will no longer have a .withDrawerContentView option. However, DocumentCameraViewableV2 and FaceCameraViewableV2 now will have the DrawerContentViewableV2 view, that you can easily override.
public var drawerContentView: DrawerContentViewableV2 = {
let view = DrawerContentViewV2(frame: .zero)
view.translatesAutoresizingMaskIntoConstraints = false
return view
}()
@objc private func setupConstraints() {
...
setupDrawerView()
}
func setupDrawerView() {
addSubview(drawerContentView)
drawerContentView.topAnchor.constraint(equalTo: topAnchor).isActive = true
drawerContentView.leftAnchor.constraint(equalTo: leftAnchor).isActive = true
drawerContentView.rightAnchor.constraint(equalTo: rightAnchor).isActive = true
drawerContentView.heightAnchor.constraint(equalToConstant: CameraSessionUIHelper.getDrawerHeight()).isActive = true
}
Also the setupUIViewFaceOval() function of FaceCameraViewV2 has been refactored with a helper function to get the drawer height:
func setupUIViewFaceOval() {
addSubview(idenfyUIViewFaceOval)
idenfyUIViewFaceOval.centerXAnchor.constraint(equalTo: centerXAnchor).isActive = true
idenfyUIViewFaceOval.bottomAnchor.constraint(equalTo: cameraSessionsButtons.idenfyUIViewContainerOfButtons.topAnchor, constant: -16).isActive = true
var top = CameraSessionUIHelper.getDrawerHeight()
// adding more for better margin
top += 16
idenfyUIViewFaceOval.topAnchor.constraint(equalTo: topAnchor, constant: top).isActive = true
idenfyUIViewFaceOval.widthAnchor.constraint(equalTo: idenfyUIViewFaceOval.heightAnchor, multiplier: 0.7).isActive = true
}
The DrawerContentViewableV2 itself has changed and will hold less views:
public protocol DrawerContentViewableV2: UIView {
var delegate: CameraSessionDrawerDelegate? { get set }
var toolbar: IdenfyToolbarV2CameraSession { get }
var infoLabel: UILabel { get }
var descriptionLabelV2: UILabel { get }
var faceDetectionAlertImage: UIImageView { get }
}
As well as the CameraSessionDrawerDelegate:
public protocol CameraSessionDrawerDelegate: AnyObject {
func backButtonPressedAction()
func toggleFlashButtonPressedAction()
func instructionDialogButtonPressedAction()
}
You can check new, fully implemented views in our sample application here
All the colors and ui customizations, related to the instructions drawer have been removed, you can check out the updated ui customization files here.
After capturing photos, camera results view will appear instantly
Since the camera results view will appear instantly, it will show a loading spinner, while the photo appears.
[8.2.x] | [8.3.0] |
---|---|
![]() | ![]() |
Please follow this migration guide point, if you have implemented custom views of CameraResultViewableV2.
The CameraResultViewableV2 now will have an additional photoResultDetailsSpinner view you must implement:
public var photoResultDetailsSpinner: LottieAnimationView = {
let lottieView = LottieAnimationView(frame: .zero)
lottieView.translatesAutoresizingMaskIntoConstraints = false
if let path = Bundle(identifier: "com.idenfy.idenfyviews")?.path(forResource: "idenfy_custom_animation_photo_result_loading_indicator", ofType: "json") {
lottieView.animation = LottieAnimation.filepath(path)
}
lottieView.contentMode = .scaleAspectFit
lottieView.play()
lottieView.loopMode = .loop
lottieView.backgroundBehavior = .pauseAndRestore
lottieView.alpha = 0.6
lottieView.isHidden = true
return lottieView
}()
Fully implemented views can be found in our sample application here
Changed photo cropping to preserve higher resolution
The position of document camera rectangle has been changed to match the logic of Android SDK. Now the x position will be 0.05 * screen width.
As well as CameraSessionsButtonsViewV2 height multiplier has been moved to a constant. It will have an effect in the picture cropping. So make sure you change the height of idenfyUIViewContainerOfButtons by changing the idenfyCameraBottomControlsHeightMultiplier constant
/**
idenfyCameraBottomControlsHeightMultiplier is used in photo cropping,
make sure you change the height of idenfyUIViewContainerOfButtons by changing the idenfyCameraBottomControlsHeightMultiplier constant
*/
open func setupUIViewContainerOfButtons() {
addSubview(idenfyUIViewContainerOfButtons)
idenfyUIViewContainerOfButtons.leadingAnchor.constraint(equalTo: leadingAnchor).isActive = true
idenfyUIViewContainerOfButtons.trailingAnchor.constraint(equalTo: trailingAnchor).isActive = true
idenfyUIViewContainerOfButtons.heightAnchor.constraint(equalTo: heightAnchor, multiplier: ConstsIdenfyUI.idenfyCameraBottomControlsHeightMultiplier).isActive = true
idenfyUIViewContainerOfButtons.bottomAnchor.constraint(equalTo: bottomAnchor).isActive = true
}
Fully implemented views can be found in our sample application here