Face authentication for Android SDK
Face Matching authentication works from 8.0.1 Android SDK versions.
Introduction
Face authentication is a perfect tool to perform KYC checks once and then use the same scanRef to perform multiple verifications just within 30 seconds!
The flow only requires the user to take a regular face photo to perform the authentication.
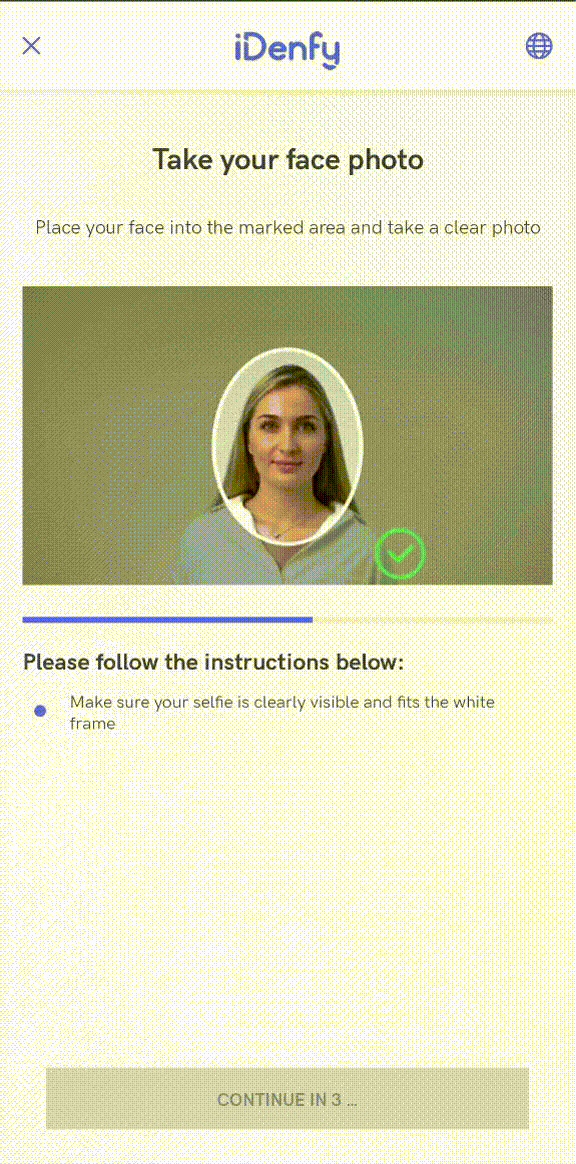
Pre-conditions
A successful verification must be performed before initializing face authentication. For face authentication all you need is scanRef, which is obtained here. Read about performing verification here.
Getting started
Follow the iDenfySDK integration guide, which is required for the face authentication as well.
After completing the steps and the application compiles successfully, you are ready to implement face-auth specific logic.
You can also download the sample app, it supports face authentication, so it should be much easier. Download here.
Follow the information below to get started.
1. Handle webhook callback
You will receive a webhook callback if it is a preferred way of handling results (it should be since it is more secure and reliable). The webhook structure is the following:
{
"token": "token",
"clientId": "clientId",
"scanRef": "scanRef",
"status": "SUCCESS",
"type": "AUTHENTICATION",
"method": "FACE_MATCHING",
"facePhoto": "https://s3.eu-west-1.amazonaws.com/production.users.storage/users_storage/users/<HASH>/FRONT.png?AWSAccessKeyId=<KEY>&Signature=<SIG>&Expires=<STAMP>"
}
The "facePhoto" key type: String (URL), Can be null: Yes, Constraints: Max length 500, Explanation: A URL to download selfie photo with which a client has completed a face authentification.
The status key has the following values:
Name | Description |
---|---|
SUCCESS | The user completed face authentication flow and the authentication status, provided by an automated platform, is SUCCESS. |
FAILED | The user completed face authentication flow and the authentication status, provided by an automated platform, is FAILED. |
CANCELED | The user did not complete the face authentication flow and canceled it and the identification status, provided by an automated platform, is EXIT. |
EXPIRED | The user did not complete the face authentication flow, but did not cancel it explicitly and the identification status, provided by an automated platform, is EXPIRED. |
To set your webhook URL, contact at tech support via our dashboard.
2. Handle callback in SDK
If you also want to handle results directly in mobile app, you can implement the result handling in the SDK as well.
private var identificationResultsCallback = registerForActivityResult(
ActivityResultContracts.StartActivityForResult()
) { result: ActivityResult ->
val resultCode = result.resultCode
val data = result.data
if (resultCode == IdenfyController.IDENFY_FACE_AUTHENTICATION_RESULT_CODE) {
val faceAuthenticationResult: FaceAuthenticationResult =
data!!.getParcelableExtra(IdenfyController.IDENFY_FACE_AUTHENTICATION_RESULT)!!
Toast.makeText(this, "Face Authentication Status: " + faceAuthenticationResult.faceAuthenticationStatus.status, Toast.LENGTH_SHORT).show()
when (faceAuthenticationResult.faceAuthenticationStatus) {
FaceAuthenticationStatus.SUCCESS -> {
// The user completed authentication flow, was successfully authenticated
}
FaceAuthenticationStatus.FAILED -> {
// The user completed authentication flow, was not successfully authenticated
}
FaceAuthenticationStatus.EXIT -> {
// The user did not complete authentication flow
}
}
}
}
3. Check face authentication status and generate the token
Before initializing the SDK, you need to check whether the user can use face authentication and generate an authToken. To do this, please head to Token Generation for SDK documentation.
4. Initialize SDK
Now all you need is to pass the generated token. Congrats! 🥳
val faceAuthenticationInitialization = FaceAuthenticationInitialization(token)
IdenfyController.getInstance().initializeFaceAuthenticationSDKV2(requireActivity(), (requireActivity() as BaseActivity).identificationResultsCallback, faceAuthenticationInitialization)
Customization
Immediate redirect
An additional bool can be passed to the function to set the immediate redirect feature. This boolean sets whether the results from iDenfy SDK should be received immediately without any additional result pages.
The client does the verification, loading state appears and closes - without showing the final status screen.
To enable it change the initialization of FaceAuthenticationInitialization in a following way:
val faceAuthenticationInitialization = FaceAuthenticationInitialization(token, true)
Face detection
Face detection feature can be enabled, which requires users to place their face into the marked area before taking a photo.
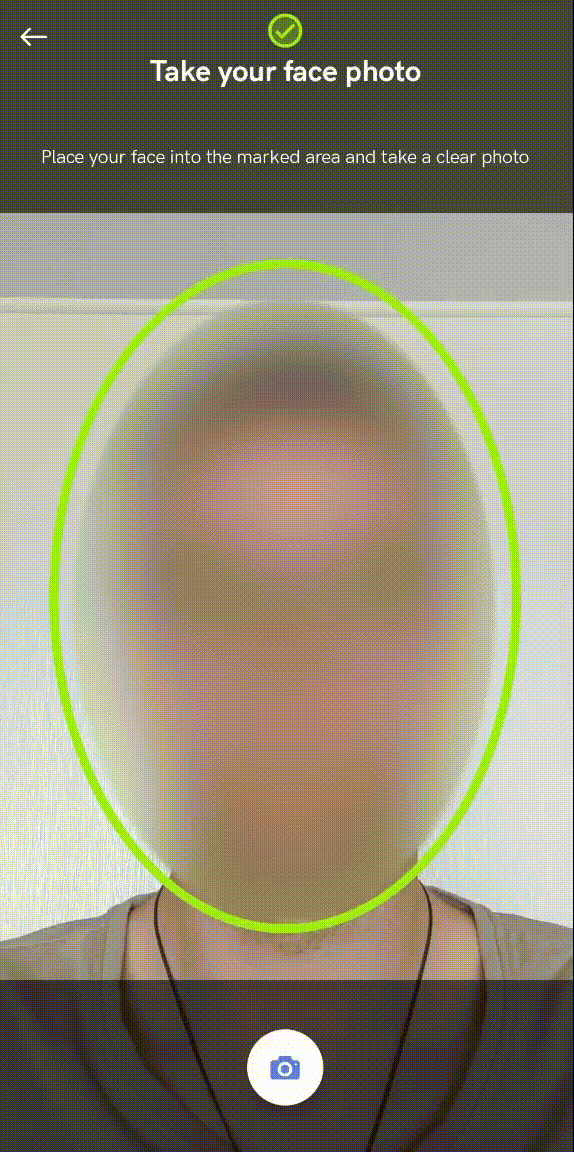
Contact our tech support via Jira customer portal for enabling this feature. Note that face detection will apply to both KYC verification and face authentication flows.
Passive liveness detection
Additionally, while using face matching authentication, passive liveness detection feature can be enabled, to detect whether a seflie from the photo is genuine or not.
Contact our tech support via Jira customer portal for enabling this feature. Note that face detection will apply to both KYC verification and face authentication flows.
Auto capture
Auto capture feature can be enabled, which requires users to place their face into the marked area and the picture will be automatically taken as well as immediately processed:
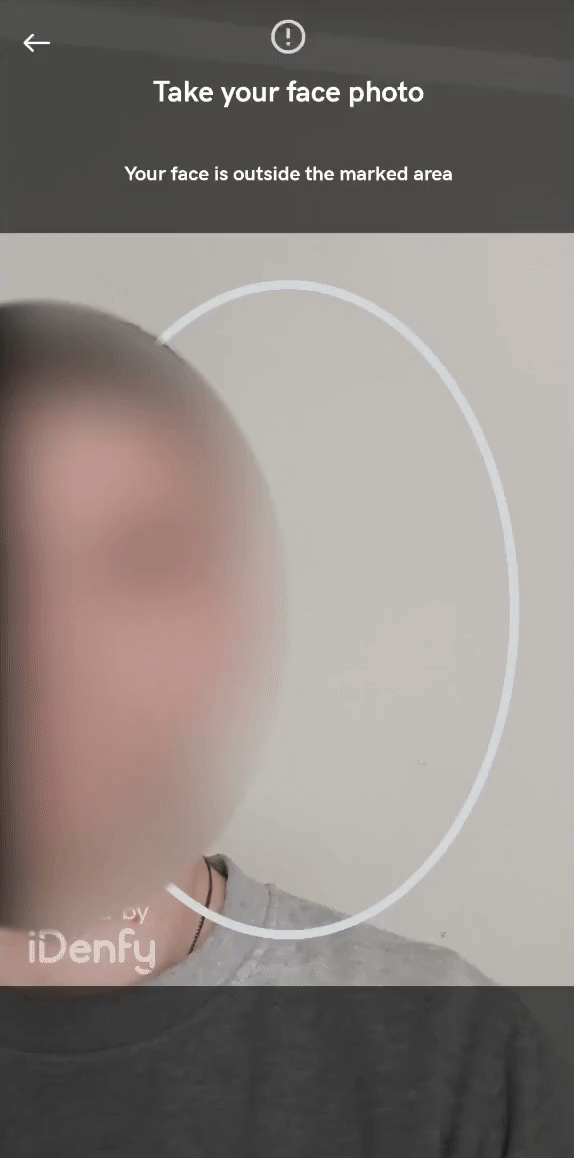
Contact our tech support via Jira customer portal for enabling this feature for face authentication.
UI Customization
The UI can be customized same as the KYC verification flow. Main colors or styles in the styles.xml or colors.xml files of your app target can be overridden, as well as the layouts.
Our colors, styles and layouts can be found in our repository.
Face authentication flow has additional UI settings, they can be passed using the IdenfyFaceAuthUIBuilder along with FaceAuthenticationInitialization:
val idenfyFaceAuthUISettings = IdenfyFaceAuthUISettings.IdenfyFaceAuthUIBuilder()
//Show or hide language selection button
.withLanguageSelection(true)
//Show or skip camera on boarding screen
.withOnBoardingView(true)
.build()
val faceAuthenticationInitialization = FaceAuthenticationInitialization(
token,
idenfyFaceAuthUISettings = idenfyFaceAuthUISettings
)